Updating constraints on the storyboard can be easy with these tips:
Enjoy this info? Visit my Patreon page to support educators and artists.
My YouTube Channel
Need help with SwiftUI? Check out my products at: Big Mountain Studio Website
Monday, March 27, 2017
Sunday, March 26, 2017
Transparent TableView - Zelda App (iOS, Xcode 8, Swift 3)
Learn how to make your table view transparent so you can see the background through it. You will also learn a little about customizing the tableview's separator.
Closures - Zelda App (iOS, Xcode 8, Swift 3)
You may not be creating closures all the time but it is good to know about them and how they work because you will be using them ALL the time.
Wednesday, March 22, 2017
Play A Movie That Is In Your Project
On your Storyboard, add a "AVKit Player View Controller". Connect it with a Segue from a UIButton you put on the View Controller.
Like this:
(Xcode 8.2, Swift 3)
Like this:
import UIKit import AVKit import AVFoundation class ViewController: UIViewController { override func prepare(for segue: UIStoryboardSegue, sender: Any?) { let destination = segue.destination as! AVPlayerViewController let url = Bundle.main.url(forResource: "myMovieName", withExtension: "m4v") if let movieURL = url { destination.player = AVPlayer(url: movieURL) } } }
(Xcode 8.2, Swift 3)
Saturday, March 18, 2017
Introduction to iOS Threading - Zelda App (Xcode 8, Swift 3)
Get an introduction to threading in iOS to make your apps more responsive when another process is taking a long time.
Sunday, March 12, 2017
Show Execution Time (Elapsed Time)
Sometimes you want to see how long it took some code to execute. Here is an example of one way you could do this.
(Swift 3)
func myLongRunningFunction() { let start = Date() // Do you work let end = Date() let elapsedTime = end.timeIntervalSince(start) print("Elapsed Time: \(elapsedTime)") }
(Swift 3)
Friday, March 10, 2017
iOS Tinder-Like Swipe - Part 5 - Scaling the Card (Xcode 8, Swift 3)
The final part! As you slide the card closer to the edge of the screen, we also want to scale the card down (make smaller). Here is how you do that:
iOS Tinder-Like Swipe - Part 4 - Rotating the Card (Xcode 8, Swift 3)
As you swipe the card side-to-side, we want to apply a rotation transform to the card. The closer you get to the edge, the more rotation. I walk you through all the steps.
iOS Tinder-Like Swipe - Part 3 - Animating card off screen (Xcode 8, Swi...
When the card gets close to the edge of the screen, we want it to continue off the screen by animating it off.
iOS Tinder-Like Swipe - Part 2 - Fading image in and out (Xcode 8, Swift 3)
Show the thumbs up or thumbs down while doing the Tinder-like swiping action on the card.
Monday, March 6, 2017
iOS Tinder-Like Swipe - Part 1- UIPanGestureRecognizer (Xcode 8, Swift 3)
Create Tinder-like swiping in your app. This was a fun tutorial series to make. Hope you enjoy it!
Also, a viewer pointed out something that can make the dragging of the card code a little simpler.
Instead of:
You can just do:
Also, a viewer pointed out something that can make the dragging of the card code a little simpler.
Instead of:
let point = sender.translation(in: view) card.center = CGPoint(x: view.center.x + point.x, y: view.center.y + point.y)
You can just do:
card.center = sender.location(in: view)Easy!
Thursday, March 2, 2017
Storyboard Tips - Easily Work With Layers Of Controls (iOS, Xcode 8)
Storyboard Tips. When working with layers of views and other controls, use this is a handy tip to get underneath other controls to make modifications and put everything back to the way it was with a click of a button!
Wednesday, March 1, 2017
Timer in Apps (Swfit 3, Xcode 8, iOS)
In this example I start a timer as soon as I enter the second view controller after clicking the "START MY RANDOM WORKOUT" button. Here is the code for that second view:
(Swift 3)
import UIKit class ExerciseVc: UIViewController { @IBOutlet weak var timerLabel: UILabel! @IBOutlet weak var icon: UIImageView! var timer: Timer! var timeLeft = 60 override func viewDidLoad() { super.viewDidLoad() // Call setTimeLeft every 1 second timer = Timer.scheduledTimer(timeInterval: 1, target: self, selector: #selector(self.setTimeLeft), userInfo: nil, repeats: true) icon.image = #imageLiteral(resourceName: "situp") } func setTimeLeft() { timeLeft -= 1 // Subtract one second if timeLeft == 0 { stop() } timerLabel.alpha = 1 timerLabel.transform = CGAffineTransform(scaleX: 1.4, y: 1.4) UIView.animate(withDuration: 1, animations: { self.timerLabel.text = "\(self.timeLeft)" self.timerLabel.alpha = 0.3 self.timerLabel.transform = .identity }) } @IBAction func stop(_ sender: UIButton) { stop() } // Show a white status bar instead of the default black one override var preferredStatusBarStyle: UIStatusBarStyle { get { return UIStatusBarStyle.lightContent } } func stop() { timer.invalidate() // Stop the timer dismiss(animated: true, completion: nil) } }
(Swift 3)
Subscribe to:
Posts (Atom)
SwiftUI Search & Filter with Combine - Part 3 (iOS, Xcode 13, SwiftUI, 2...
In part 3 of the Searchable video series, I show you how to use Combine in #SwiftUI for the search and filter logic connected to the searcha...
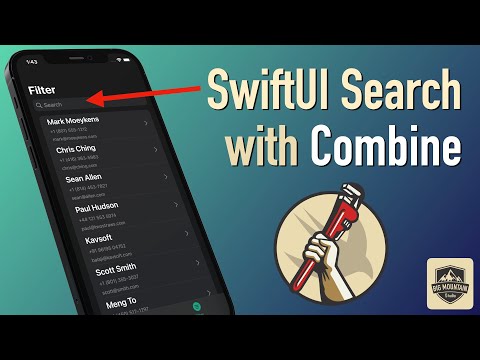
-
I had someone ask about aligning text within a TextField view and I didn't have a page in my book for this but added one just now. ...
-
I couldn't find a tutorial on this so this is my documented journey into trying to figure out how to implement OAuthSwift into an iOS p...
-
In this example I use a UIToolBar to contain the done button but you can use any UIView to assign to inputAccessoryView . class ViewCont...