Enjoy this info? Visit my Patreon page to support educators and artists.
My YouTube Channel
Need help with SwiftUI? Check out my products at: Big Mountain Studio Website
Monday, January 19, 2015
Control Flow
If
if myFirstName == "Mark"{ print("Hello, \(myFirstName). You are an admin.") } else { print("Welcome, guest!") }Ternary Conditional Operator
let isAdmin: Bool = myFirstName == "Mark" ? true : falseSwitch
switch myFirstName { case "Mark": print("Hello, \(myFirstName). You are an admin.") case "Mark", "Chase": print("Hello, \(myFirstName). You are a user.") default: print("Welcome, guest!") } // Note: No "break" statement is necessary.For In
let iterations = 5 for index in 1...iterations { print("This is iteration \(index)") } // Note: The for loop is kind of deprecated, replaced by the for in loop. for var x = 0; x < iterations; ++x { print("This is iteration \(x)") } For more examples of for in loops see post on CollectionsWhile
var x = 0 while x < 5 { print("This is iteration \(x)") x++ } repeat { print("This is iteration \(x)") x++ } while x < 5 (Updated for Swift 2.2)Member Declarations
Variable Members
var myFirstName = "Mark" // Implicit - Will make variable a string var myLastName: String = "Moeykens" // Explicit var accountBalance: Float = 5012.123456 var measure: Double = 1.123456789012345 var isBlogging = true // Implicitly converts "isBlogging" to booleanConstant Members
let thing = "tree" thing = "stone" // This is invalid
Subscribe to:
Posts (Atom)
SwiftUI Search & Filter with Combine - Part 3 (iOS, Xcode 13, SwiftUI, 2...
In part 3 of the Searchable video series, I show you how to use Combine in #SwiftUI for the search and filter logic connected to the searcha...
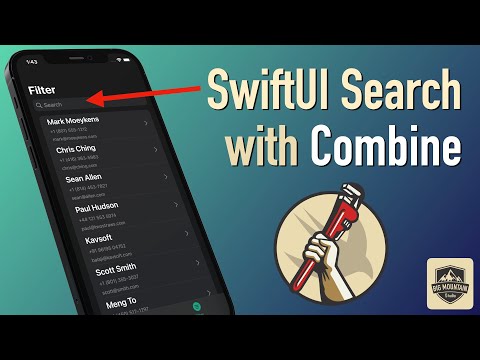
-
I had someone ask about aligning text within a TextField view and I didn't have a page in my book for this but added one just now. ...
-
I couldn't find a tutorial on this so this is my documented journey into trying to figure out how to implement OAuthSwift into an iOS p...
-
In this example I use a UIToolBar to contain the done button but you can use any UIView to assign to inputAccessoryView . class ViewCont...