Learn how to use UserDefaults to save data in your app.
Enjoy this info? Visit my Patreon page to support educators and artists.
My YouTube Channel
Need help with SwiftUI? Check out my products at: Big Mountain Studio Website
Monday, January 30, 2017
Sunday, January 29, 2017
How to make an Onboarding Screen (iOS, Xcode 8, Swift 3)
Show an onboarding (intro) screen the first time the user uses your app. Keep track if they saw it or not so you know if it should come first.
Wednesday, January 25, 2017
How to Animate a Button Menu (iOS, Xcode 8, Swift 3)
Animate this cool button menu using UIView.animate function.
Friday, January 20, 2017
How to Customize the UISlider (iOS, Xcode 8, Swift 3)
There is a lot you can do to improve how the default UISlider looks! Watch this video to learn how.
Radial Gradients on a UIView (iOS, Xcode 8, Swift 3)
Radial gradients were a lot harder in iOS (Swift) than just normal gradients.
Monday, January 2, 2017
Gradients
Below is a simple gradient from black to dark gray. You can add many colors to the colors array.
You can manually set the startPoint and endPoint properties for the CAGradientLayer to change the default gradient direction from top to bottom.
These are of a type CGPoint which is basically just and x and y value. BUT, the max value for x or y is only 1.
You have to think of the values as a percentages, like:
If you wanted to make it HORIZONTAL, then you could start from upper left to upper right.
It would look like this:
Now, can you guess what a 45 degree angle would look like?
It would look like this:
Here's a diagram which might clear up how these CGPoints work:
(Swift 3)
let newLayer = CAGradientLayer() newLayer.colors = [UIColor.black.cgColor, UIColor.darkGray.cgColor] newLayer.frame = view.frame view.layers.insertSublayer(newLayer, at: 0)
You can manually set the startPoint and endPoint properties for the CAGradientLayer to change the default gradient direction from top to bottom.
These are of a type CGPoint which is basically just and x and y value. BUT, the max value for x or y is only 1.
You have to think of the values as a percentages, like:
- 0 = 0%
- .25 = 25%
- .5 = 50%
- .75 = 75%
- 1 = 100%
newLayer.startPoint = CGPoint(x: 0, y: 0) // Upper left corner
newLayer.endPoint = CGPoint(x: 0, y: 1) // Lower left corner
If you wanted to make it HORIZONTAL, then you could start from upper left to upper right.
It would look like this:
newLayer.startPoint = CGPoint(x: 0, y: 0) // Upper left corner
newLayer.endPoint = CGPoint(x: 1, y: 0) // Upper right corner
Now, can you guess what a 45 degree angle would look like?
It would look like this:
newLayer.startPoint = CGPoint(x: 0, y: 0) // Upper left corner
newLayer.endPoint = CGPoint(x: 1, y: 1) // Lower right corner
Here's a diagram which might clear up how these CGPoints work:
![]() |
CGPoint X,Y Scale in iOS |
(Swift 3)
Subscribe to:
Posts (Atom)
SwiftUI Search & Filter with Combine - Part 3 (iOS, Xcode 13, SwiftUI, 2...
In part 3 of the Searchable video series, I show you how to use Combine in #SwiftUI for the search and filter logic connected to the searcha...
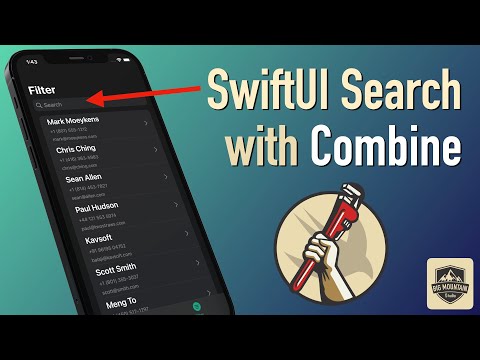
-
I had someone ask about aligning text within a TextField view and I didn't have a page in my book for this but added one just now. ...
-
I couldn't find a tutorial on this so this is my documented journey into trying to figure out how to implement OAuthSwift into an iOS p...
-
In this example I use a UIToolBar to contain the done button but you can use any UIView to assign to inputAccessoryView . class ViewCont...